What is python?
Python is an Object-Oriented Programming Language.
What is OOP or Object-Oriented programming?
Object-Oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviours into individual objects.
Features of Python:
- Python is freely available at the official website & one can easily download it. So, it is an open source.
- Although python is a open high level language, coding in python is easy as compared to other languages like Java, Cobol, C++, etc and one can learn its basics within a few days.
- Python is used for scientific & non-scientific programming.
Advantages of Python:
- It is easy to learn.
- It is platform independent and can run across different platforms like Windows, Mac, Linux/Unix, etc.
- It is an interactive, interpreted and Object-Oriented Programming language.
Python Character Set
Character set is a set of valid characters recognized by python. A character represents any letter, digit, or any other symbol. It uses the traditional ASCII character set.
Python supports the following character sets:
- Letters: A-Z, a-z
- Digits: 0-9
- Special Symbols: +, -, *, /, (), [], etc.
- Write Spaces: Blank Space, tabs (->), newline, etc.
Tokens
A token is the smallest element of a python script that is meaningful to the interpreter.
The following categories of tokens exists: Identifiers, Keywords, literals, operators, and delimiters.
1) Identifiers
The name of any variable, constant, function or module is called an identifier.
Example: – X12Y, India_123, Swap, etc.
2) Literals
A fixed numeric or non-numeric value is called a literal. It can be defined as a number, text, or other data that represents values to be stored in a variable.
Example: – 2, -23.6, “abc”, etc.
3) Keywords:
The reserved words of Python. Which have a special fixed meaning for the interpreter are called keywords.
Example: – int, float, True, False, and, break, etc.
Note: – There are 33 keywords in Python.
Operators
The Symbols or words that performs some kind of operation on a given value and returns the results are called operators.
There are three types of operators:
- Arithmetic Operators
- Relational Operators
- Logical Operators
Arithmetic Operators
Arithmetic Operators are used to perform mathematical operations like, addition, subtraction, multiplication, and division.
SL No. | Symbol | Description | Example |
1 | + | Addition | i) 55+45 ii) ’Good ’+’Morning’ |
2 | – | Subtraction | 10-15 |
3 | * | Multiplication | 3*2 |
4 | / | Division | 17/5 |
5 | % | Modulus | 10%2 |
6 | ** | Exponential | 3**2 |
7 | // | Integer division | 9//2 |
Relational Operators
Relational Operators are used for comparing two expressions and results in either True or False.
SL No. | Symbol | Description | Example |
1 | < | Less than | 7<10 True 110<20 False |
2 | > | Greater than | 7>5 True 10>10 False |
3 | <= | Less than equal to | 2<=5 True 7<=4 False |
4 | >= | Greater than equal to | 10>=10 True 10>=12 False |
5 | == | Equal to | 10==10 True 10==11 False |
6 | !=, <> | Not equal to | 10!=11 True 10!=10 False |
Unary/Binary Operator
An operator can be termed as unary or binary depending upon the number of operands it takes. An unary operator takes only one operand and a binary operator takes two operands.
Logical Operators
Logical Operators are used to perform logical operations on the values of values of variables.
SL No. | Symbol | Description | Example |
1 | And | If anyone of the operands is true, then the condition become true. | True and True True True and False False |
2 | Or | If anyone of the operands is true, then the condition become true. | True and False True False and False False |
3 | Not | Reverses the state of operand/condition | not True False not False True |
Programs
1) Write a program in python to print the sum of two numbers.
X=10 Y=20 Z=X+Y print(“The sum is :”,Z)
Output
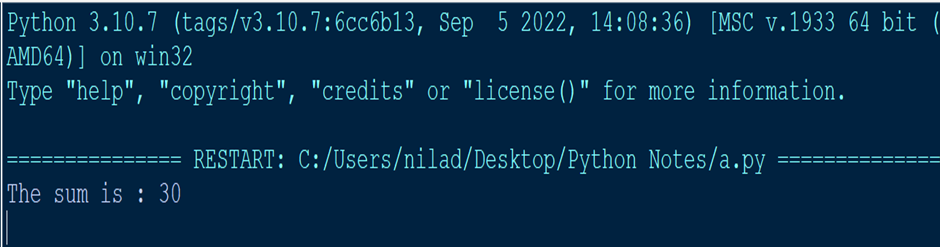
2) Write a program in python to print the sum, difference, multiplication & division.
X=10 Y=20 Z=X+Y A=X-Y B=X*Y C=X/Y print(“The sum is :”,Z) print(“The difference is :”,A) print(“The Product is :”,B) print(“The Division is :”,C)
Output
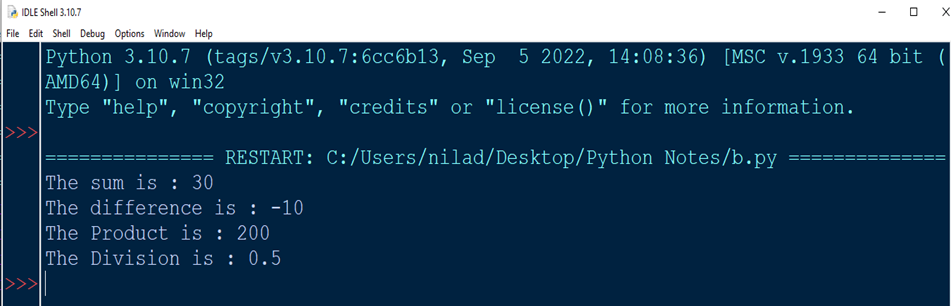
3) Write a program in python to take two numbers as input & print their sum.
X=int(input(“Enter 1st Number”)) Y=int(input(“Enter 2nd Number”)) Z=X+Y print(“The sum is :”,Z)
Output
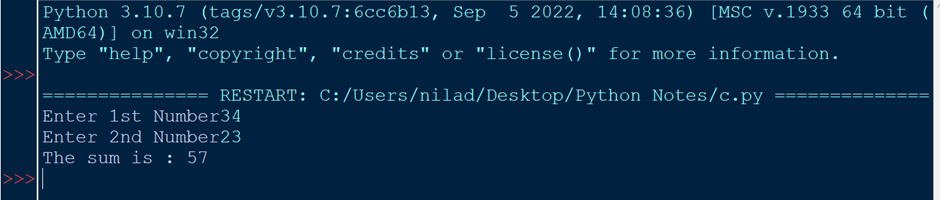